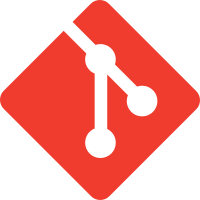
This is a rather large commit that includes all of the following: - React UI with code editor, runtime renderer and input-output panes - Language providers for a sample language and Brainfuck - Implementation of code execution in a web worker - All-at-once unabortable execution of program fully functional
34 lines
996 B
TypeScript
34 lines
996 B
TypeScript
import { TextArea } from "@blueprintjs/core";
|
|
|
|
/**
|
|
* For aesthetic reasons, we use readonly textarea for displaying output.
|
|
* Textarea displays placeholder if value passed is empty string, which is undesired.
|
|
* This function is a fake-whitespace workaround.
|
|
*
|
|
* @param value Value received from parent. Placeholder shown on `null`.
|
|
* @returns Value to pass as prop to Blueprint TextArea
|
|
*/
|
|
const toTextareaValue = (value: string | null): string | undefined => {
|
|
if (value == null) return undefined; // Placeholder shown
|
|
if (value === "") return "\u0020"; // Fake whitespace to hide placeholder
|
|
return value; // Non-empty output value
|
|
};
|
|
|
|
type Props = {
|
|
value: string | null;
|
|
};
|
|
|
|
export const OutputViewer = ({ value }: Props) => {
|
|
return (
|
|
<TextArea
|
|
fill
|
|
large
|
|
readOnly
|
|
growVertically
|
|
value={toTextareaValue(value)}
|
|
placeholder="Run code to see output..."
|
|
style={{ height: "100%", resize: "none", boxShadow: "none" }}
|
|
/>
|
|
);
|
|
};
|