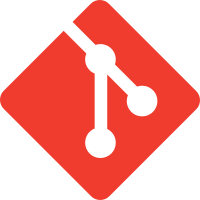
This is a rather large commit that includes all of the following: - React UI with code editor, runtime renderer and input-output panes - Language providers for a sample language and Brainfuck - Implementation of code execution in a web worker - All-at-once unabortable execution of program fully functional
39 lines
859 B
TypeScript
39 lines
859 B
TypeScript
import React from "react";
|
|
import { TextArea } from "@blueprintjs/core";
|
|
|
|
// Interface for interacting with the editor
|
|
export interface InputEditorRef {
|
|
/**
|
|
* Get the current text content of the editor.
|
|
*/
|
|
getValue: () => string;
|
|
}
|
|
|
|
/**
|
|
* A very simple text editor for user input
|
|
*/
|
|
const InputEditorComponent = (_: {}, ref: React.Ref<InputEditorRef>) => {
|
|
const textareaRef = React.useRef<HTMLTextAreaElement | null>(null);
|
|
|
|
React.useImperativeHandle(
|
|
ref,
|
|
() => ({
|
|
getValue: () => textareaRef.current!.value,
|
|
}),
|
|
[]
|
|
);
|
|
|
|
return (
|
|
<TextArea
|
|
fill
|
|
large
|
|
growVertically
|
|
inputRef={textareaRef}
|
|
placeholder="Enter program input here..."
|
|
style={{ height: "100%", resize: "none", boxShadow: "none" }}
|
|
/>
|
|
);
|
|
};
|
|
|
|
export const InputEditor = React.forwardRef(InputEditorComponent);
|