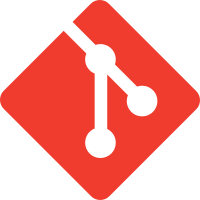
This is a rather large commit that includes all of the following: - React UI with code editor, runtime renderer and input-output panes - Language providers for a sample language and Brainfuck - Implementation of code execution in a web worker - All-at-once unabortable execution of program fully functional
59 lines
1.4 KiB
TypeScript
59 lines
1.4 KiB
TypeScript
import React from "react";
|
|
import { Mosaic, MosaicNode, MosaicWindow } from "react-mosaic-component";
|
|
|
|
// IDs of windows in the mosaic layout
|
|
type WINDOW_ID = "editor" | "renderer" | "input" | "output";
|
|
|
|
const WindowTitles = {
|
|
editor: "Code Editor",
|
|
renderer: "Visualization",
|
|
input: "User Input",
|
|
output: "Execution Output",
|
|
};
|
|
|
|
type Props = {
|
|
renderEditor: () => React.ReactNode;
|
|
renderRenderer: () => React.ReactNode;
|
|
renderInput: () => React.ReactNode;
|
|
renderOutput: () => React.ReactNode;
|
|
};
|
|
|
|
export const MainLayout = (props: Props) => {
|
|
const MOSAIC_MAP = {
|
|
editor: props.renderEditor,
|
|
renderer: props.renderRenderer,
|
|
input: props.renderInput,
|
|
output: props.renderOutput,
|
|
};
|
|
|
|
const INITIAL_LAYOUT: MosaicNode<WINDOW_ID> = {
|
|
direction: "row",
|
|
first: "editor",
|
|
second: {
|
|
direction: "column",
|
|
first: "renderer",
|
|
second: {
|
|
direction: "row",
|
|
first: "input",
|
|
second: "output",
|
|
},
|
|
},
|
|
};
|
|
|
|
return (
|
|
<Mosaic<keyof typeof MOSAIC_MAP>
|
|
className="mosaic-blueprint-theme bp3-dark"
|
|
initialValue={INITIAL_LAYOUT}
|
|
renderTile={(windowId, path) => (
|
|
<MosaicWindow<number>
|
|
path={path}
|
|
title={WindowTitles[windowId]}
|
|
toolbarControls={<span />}
|
|
>
|
|
{MOSAIC_MAP[windowId]()}
|
|
</MosaicWindow>
|
|
)}
|
|
/>
|
|
);
|
|
};
|